Overview
Codeducator is a desktop address book application for private programming language tutors.
Users are able to track the progress of their students, manage their tutoring schedule and other important information about their students.
Codeducator has a graphical user interface built with JavaFX but most of the user interactions are done using command line interface. It is written in Java and has about 10 kLoC.
This project is based on the AddressBook-Level4 created by the SE-EDU initiative.
Summary of contributions
-
Major enhancement: added a separate profile page for students and the ability to edit their miscellaneous details.
-
What it does:
-
The profile page allows the tutor to view a student’s full information paticulars on a separate page.
-
This profile page includes the main information of a student (also displayed on the student card), miscellaneous information and profile picture (both not displayed on the student card)
-
The tutor will be able to edit all of the student’s information displayed on the profile page. This includes the profile picture which is done by proving a filepath to the new picture.
-
-
Justification: A tutor would wish to learn as much details as he/she can of a student before taking one in. In the Codeducator app, there is a panel of cards to display each student’s information. However, it is relatively small. Moreover, some details like Next-Of-Kin contact is important, yet not required very often. Thus this enhancement aids the tutor in viewing and keeping track of the full details of each student.
-
Highlights: This feature was implemented partially using HTML and JavaScript and hence further changes to it will have to consider both the source code and the HTML/JavaScript files.
-
-
Minor enhancement: Added a 'ProgrammingLanguage' field to the student model.
-
What it does: Allows the tutor to keep track of what programming language(s) are being used or taught to each student.
-
**Justification: A tutor would want to keep track of what programming language a student is learning with or about, in order to better prepare lessons for that student.
-
Code contributed: [Functional code] [Test code]] {give links to collated code files}
-
Other contributions:
-
Project management:
-
Setup Github organisation and repositories for the team.
-
Managed release
v1.5rc
(1 release) on GitHub.
-
-
Enhancements to existing features:
-
Added Programming Language field to student model.
-
-
Documentation:
-
Corrected and improved on some language and grammar errors.
-
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Start of extract from [User Guide]
Student Profile Page
Displaying profile page for each student : moreInfo
Displays the full information of a student on the browser panel. The full information of a student consists of 3 elements, main information, miscellaneous information and his/her profile picture. You will be able to view his/her profile picture if one exists.
Format: moreInfo INDEX
The moreInfo command cannot work if there is no existing XML data of students. Should you encounter this warning,you can either:1) Simply enter clear and start out with an empty student list.2) Add, edit or delete a student using their corresponding commands to generate XML data of the students. |
Steps taken to display the profile page of a student
Suppose you wish to view the profile page of the 1st student of the current student list
-
Enter the command as shown below:
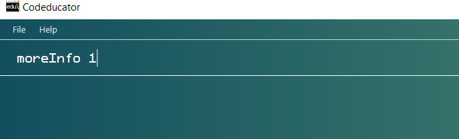
-
You will then be able to view the profile page of the student. Enter the same command with a different number for viewing the profile page of other students (e.g.
moreInfo 2
).
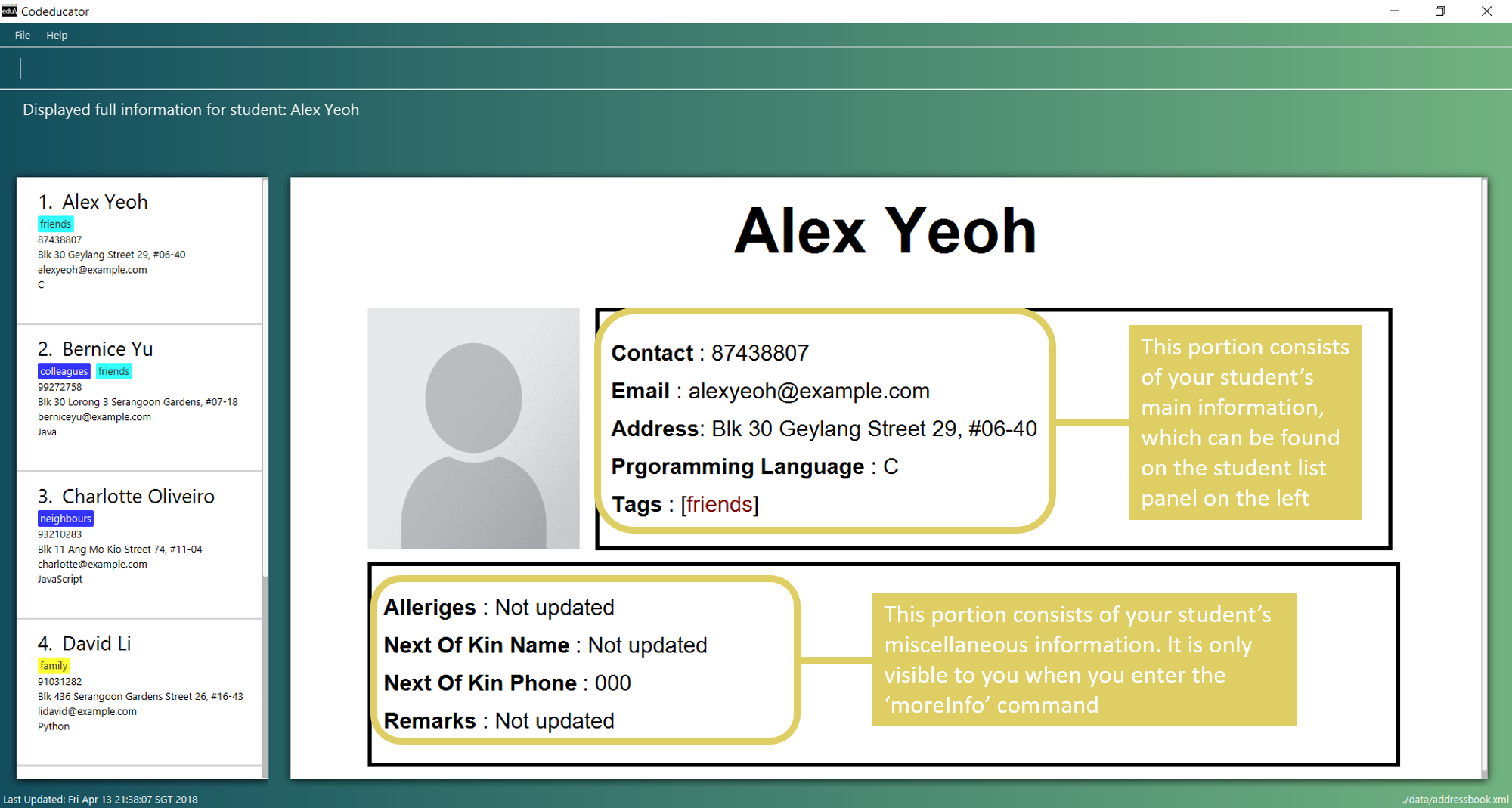
moreInfo 1
displays the 1st student (Alex Yeoh’s) profile page
You will be able to attain the profile page style in Figure 5 if your OS zoom scale is set to 125% . The profile photo may seem of a different size for other configurations. Search you computer settings should you wish to change this.
|
Suppose you wish to have this picture, with the file name of animal.jpg
as a new profile picture as your student:
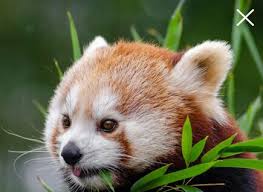
-
Enter the command as shown below:
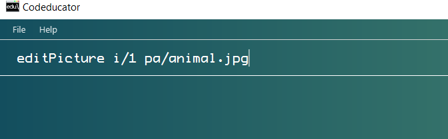
-
You will edit the profile picture of the 1st student of the latest student list to a picture existing in the same folder as the jar file with the name
animal.jpg
. You will then see the following:
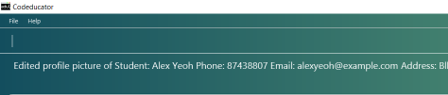
-
By calling the
moreInfo
command (found in the section above), you will be able to view the student’s profile page with the updated picture as shown below:
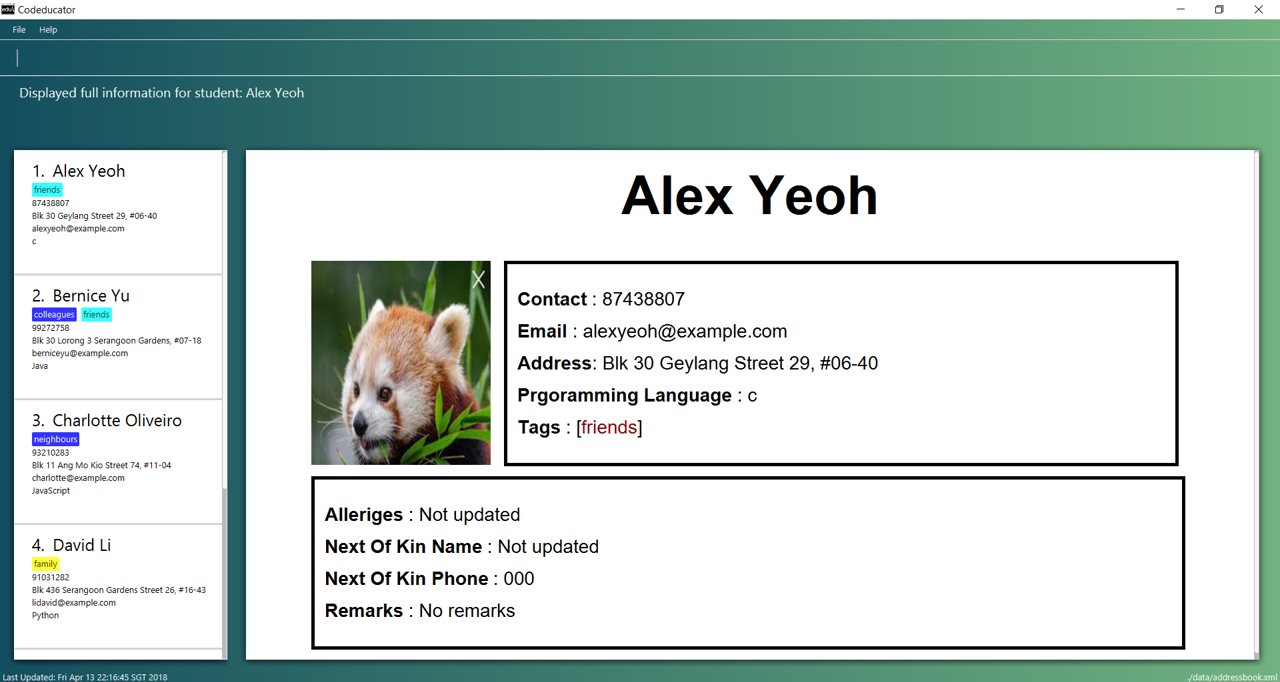
The ideal aspect ratio of the picture is 1.25 from height to width. |
End of extract from [User Guide]
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Start of extract from [Developer Guide]
A new student would then be added. On the other hand, editing a student’s programming language will be done by creating an edited student in such a way:
private static Student createEditedStudent(Student studentToEdit, EditStudentDescriptor editStudentDescriptor) {
assert studentToEdit != null;
// ...Set other attributes of the prospective newly edited student...
ProgrammingLanguage updatedProgrammingLanguage = editStudentDescriptor.getProgrammingLanguage();
return new Student(updatedName, updatedPhone, updatedEmail, updatedAddress, updatedProgrammingLanguage,
updatedTags);
}
The editedStudent will have the new programming language attribute and will hence be used to replace in the Address Book in-memory.
Design Considerations
Aspect | Alternatives | Pros (+)/ Cons(-) |
---|---|---|
How to store |
Store as an attribute of Student. (current choice) |
+ : Easy to keep track of as well as modify. |
Store as a separate list and have each student index in the UniqueStudentsList be mapped to each item in the list. |
+ : Less coupling so less need to refactor code |
|
What command to add |
Implement it through the existing |
+ : Intuitive and the user does not have to learn an additional command |
Implement it as a new command. |
+ : User will be able to add or modify |
moreInfo
Command
For the command moreInfo
, the model manager calls upon the data storage (addressbook) to raise an event for the Browser Panel to display the profile page of a student.
The moreInfo command cannot function if there is no real existing XML data of students. Thus, the sample student data provided at the initial start up will not work with this command. A warning will be mentioned if the command is called without existing data.
|
The code below shows how the the method is called with the parameter of the required Student
functions:
public void displayStudentDetailsOnBrowserPanel(Student target) throws StudentNotFoundException,
StorageFileMissingException {
addressBook.checkForStudentInAddressBook(target);
checkIfStorageFileExists();
indicateRequiredStudentIndexChange(filteredStudents.indexOf(target));
indicateBrowserPanelToDisplayStudent(target);
}
The method checkIfStorageFileExists()
checks if there is any real XML data of students at the moment. If none exists, then an exception is thrown and the command will not perform (as mentioned in the note above).
The method indicateRequiredStudentIndexChange(Index indexOfStudent)
calls the modifying of XML data of which student is needed to display his/her profile page. This is because the HTML files
can only read data from XML files and hence, an external XML file containing the index of the student whose profile page is required to be shown is needed.
Lastly, indicating the browser panel to display a student will raise a StudentInfoDisplayEvent
which is handled in the BrowserPanel
with the following code:
private void handleStudentInfoDisplayEvent(StudentInfoDisplayEvent event) {
//... logging process...
loadStudentInfoPage();
//... raising event to switch panels...
}
The diagram below shows how the event is handled in the BrowserPanel
:
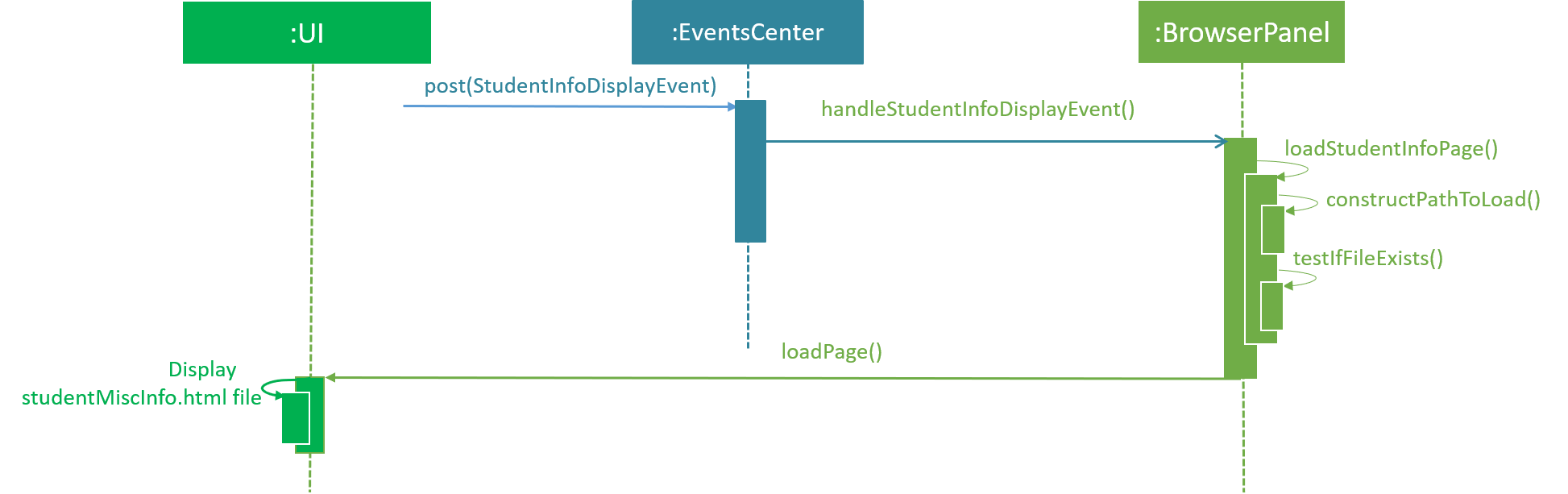
editPicture
Command
For the command editPicture
, the student’s index will have to be provided by the user again. The next parameter for this is the required file path of the picture file.
This can be in the form of an absolute file path (starting from a hardrive like C:/Users/…/picture.png
) or relative to the folder that the jar application is in.
This command uses the similar method of the edit
command and the editMisc
command where a new Student
with the edited details is created to overwrite the current existing student.
In this case, the ProfilePicturePath
of the student is edited. When this command is called, a ProfilePictureChangeEvent
will be raised and the Storage Manager will call a method to save the data of the profile picture from its original location to a location in the jar folder.
The code below shows how the saving of the file is done:
public void saveProfilePicture(ProfilePicturePath pathToChangeTo, Student student) throws IOException {
//... ensuring that the picture's filepath exists
//... getting the extension of the provided filepath of the picture
deleteExistingProfilePicture(studentPictureFilePath);
Path studentPictureFilePathWithExtension = Paths.get(studentPictureFilePath.toString() + extension);
logger.fine("Attempting to write to data file: data/" + student.getUniqueKey().toString());
Files.copy(newPath, studentPictureFilePathWithExtension);
}
Thus, the HTML file for displaying the student’s profile page will be able to show the new image, which is copied to the local jar folder.
The following sequence diagram illustrates the process of calling the editPicture
command.
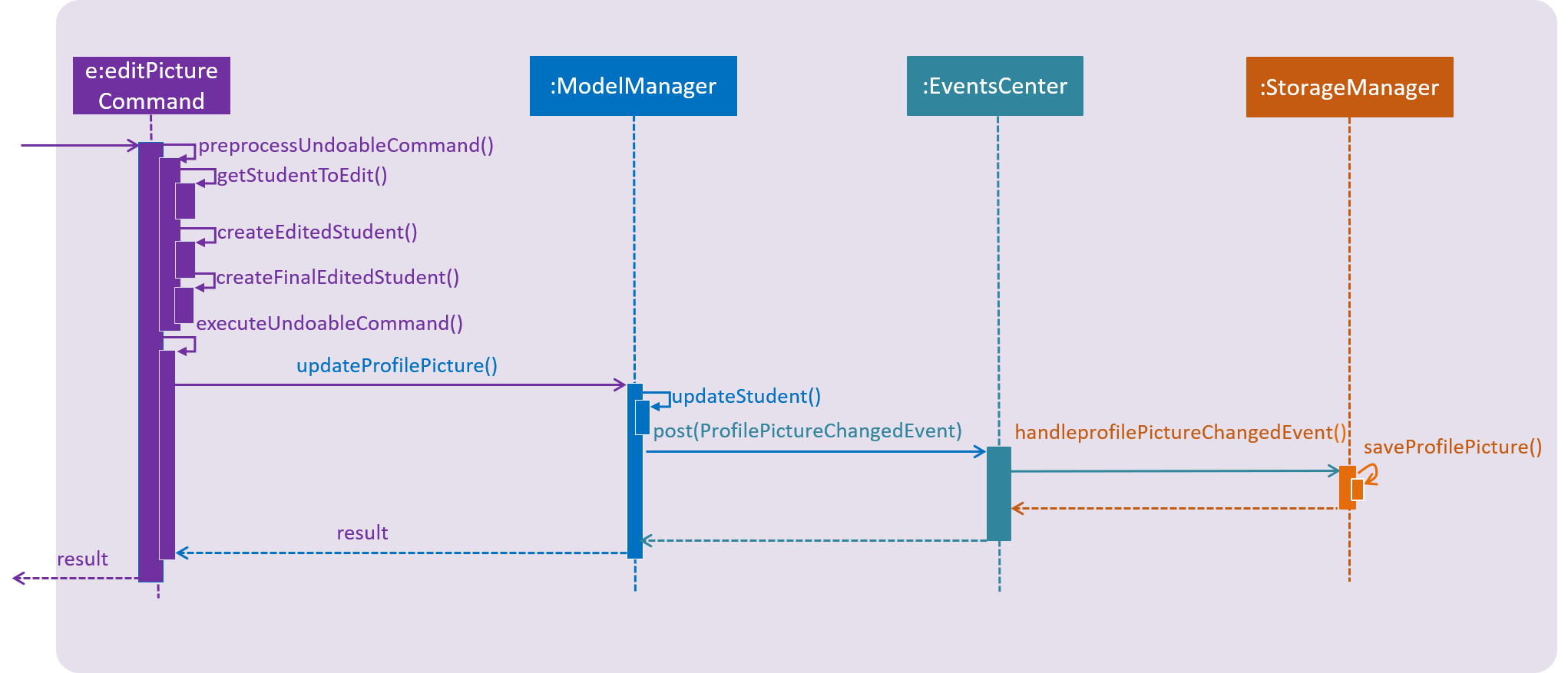
editPicture
commandEnd of extract from [Developer Guide]